App Management
Overview
The App Management API provides a way to manage io.Connect Desktop apps. It offers abstractions for:
- App - an app as a logical entity, registered in io.Connect Desktop with some metadata (name, description, icon, etc.) and with all the configuration needed to spawn one or more instances of it. The App Management API provides facilities for retrieving app metadata and for detecting when an app is added or removed.
ℹ️ For details on how to define and configure an app, see the Developers > Configuration > Application section.
- Instance - a running copy of an app hosted in an io.Connect Window. The App Management API provides facilities for starting and stopping app instances and tracking events related to app instances.
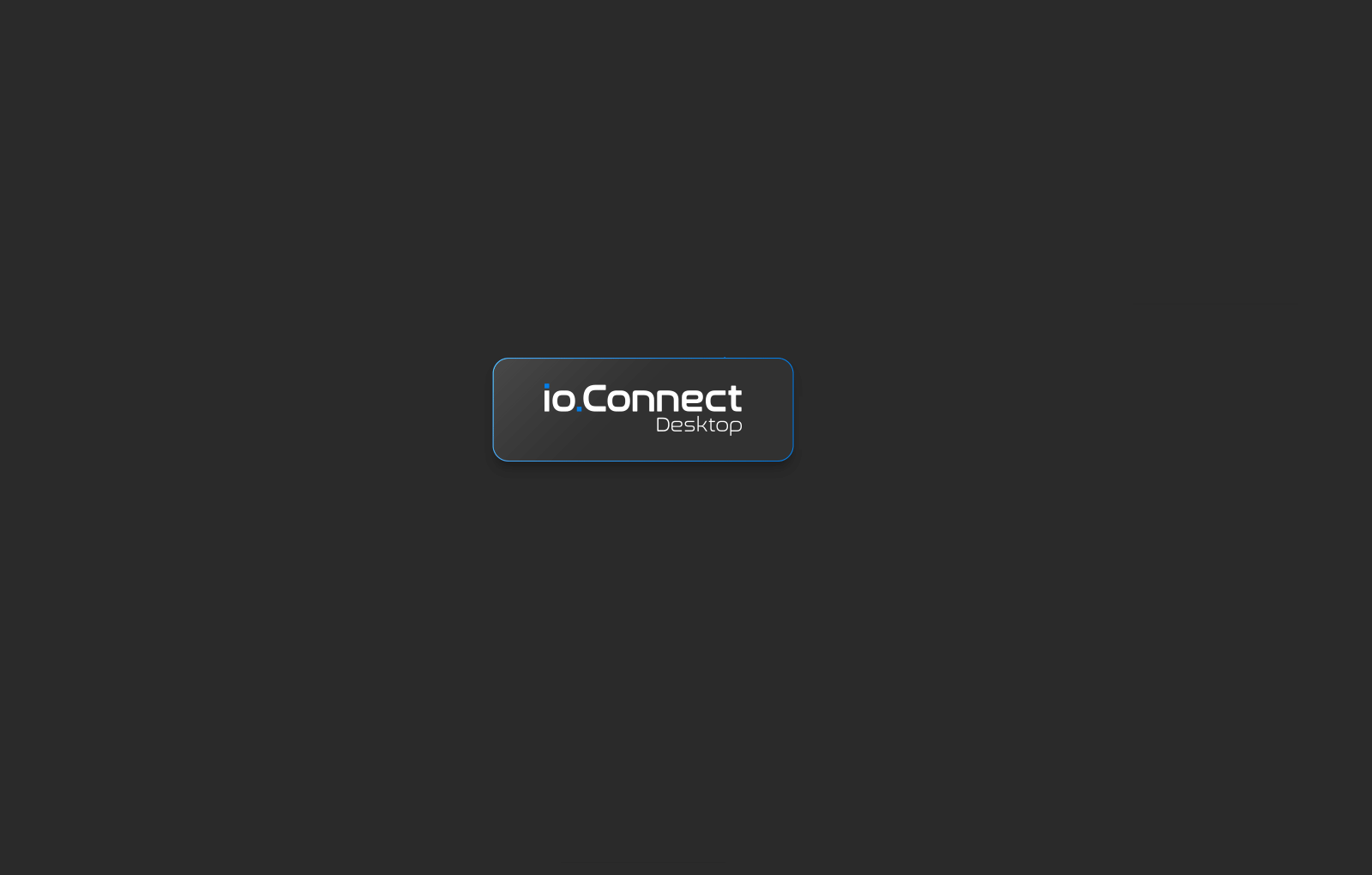
App Stores
io.Connect Desktop can obtain app definitions from a local store, from a remote REST service, or from io.Manager. To specify app stores with app definitions, use the "appStores"
top-level key in the system.json
system configuration file of io.Connect Desktop. It accepts an array of objects defining one or more app stores.
In the standard io.Connect Desktop deployment model, app definitions aren't stored locally on the user machine, but are served remotely. If io.Connect Desktop is configured to use a remote app store, it will poll it periodically and discover new app definitions. The store implementation is usually connected to an entitlement system based on which different users can have different apps or versions of the same app. In effect, io.Connect Desktop lets users run multiple versions of the same app simultaneously and allows for seamless forward/backward app rolling.
⚠️ Note that io.Connect Desktop respects the FDC3 standards and can retrieve standard io.Connect, as well as FDC3-compliant app definitions. For more details on working with FDC3-compliant apps, see the FDC3 Compliance section, the FDC3 App Directory documentation and the FDC3 Application schema.
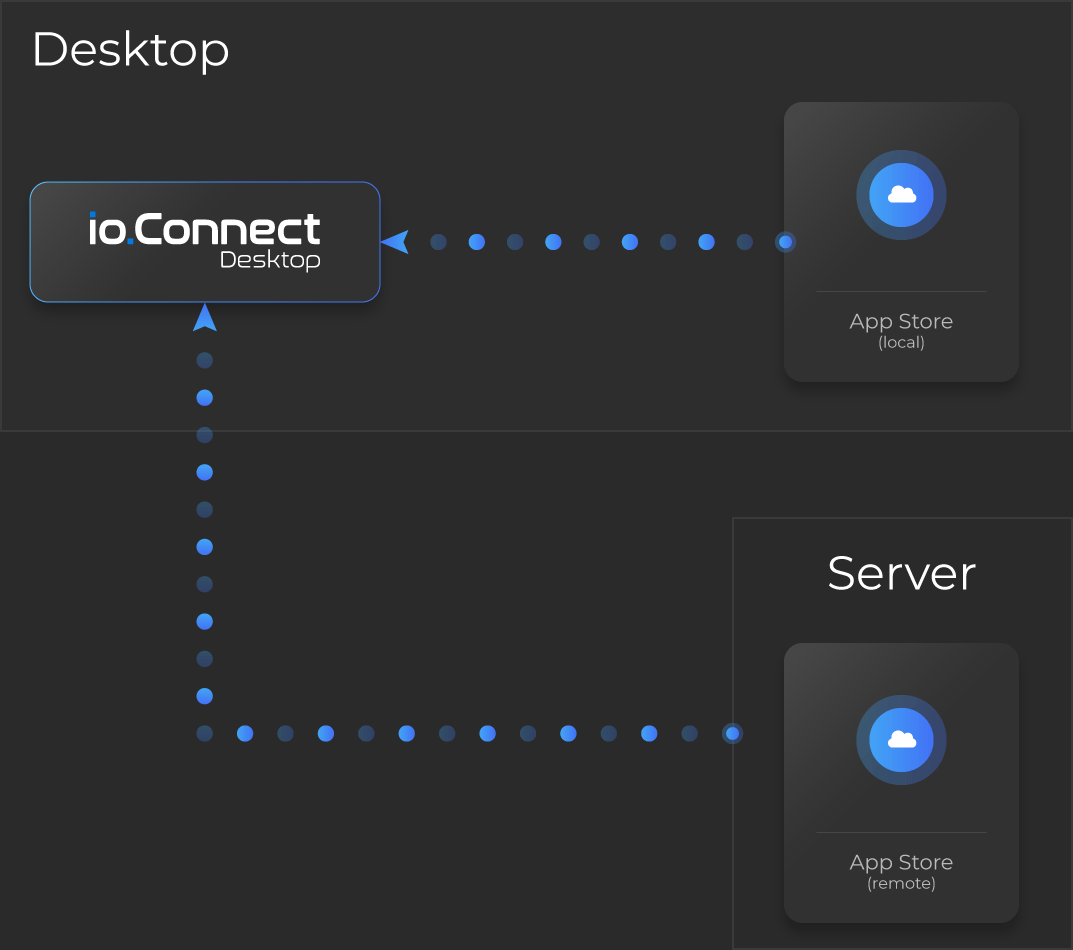
Local
To configure io.Connect Desktop to load app definition files from a local path, set the "type"
property of the app store configuration object to "path"
and specify a relative or an absolute path to the app definitions. The environment variables set by io.Connect Desktop can also be used as values:
{
"appStores": [
{
"type": "path",
"details": {
"path": "./config/apps"
}
},
{
"type": "path",
"details": {
"path": "%GLUE-USER-DATA%/apps"
}
}
]
}
Each local path app store object has the following properties:
Property | Type | Description |
---|---|---|
"appDefinitionOverrides" |
object |
Valid app definition properties that will override the ones in all app definitions from the app store. Top-level app definition properties can be specified directly in the "appDefinitionOverrides" object. The properties that are found under the "details" top-level key for each app definition type must be specified in a top-level object with the same name as the app type - "window" , "exe" , "node" , "workspaces" , "webGroup" , "clickonce" , "citrix" or "childWindow" . Available since io.Connect Desktop 9.1. |
"details" |
object |
Required. Specific details about the app store. |
"isRequired" |
boolean |
If true (default), the app store will be required. If the app store can't be retrieved, io.Connect Desktop will throw an error and shut down. If false , io.Connect Desktop will initiate normally, without apps from that store. |
"type" |
string |
Required. Type of the app store. Must be set to "path" for local path app stores. |
The "details"
object for a local path app store has the following properties:
Property | Type | Description |
---|---|---|
"path" |
string |
Required. Must point to the local app store. The specified path can be absolute or relative and you can use defined environment variables. |
REST
App definitions can also be obtained from remote app stores via a REST service.
To configure a connection to the REST service providing the remote app store, add a new entry to the "appStores"
top-level key in the system.json
file and set its "type"
to "rest"
:
{
"appStores": [
{
"type": "rest",
"details": {
"url": "https://example.com/my-app-store/",
"auth": "no-auth",
"pollInterval": 30000,
"enablePersistentCache": true,
"cacheFolder": "%LocalAppData%/interop.io/io.Connect Desktop/UserData/%GLUE-ENV%-%GLUE-REGION%/configCache/"
},
"appDefinitionOverrides": {
// Overrides for some top-level app definition properties.
"ignoreSavedLayout": true,
"allowMultiple": false,
// Overrides for the properties under the `"details"` top-level key in apps of type `"window"`.
"window": {
"autoInjectAPI": {
"enabled": true,
"autoInit": {
"channels": true
}
}
}
}
}
]
}
Each remote app store object has the following properties:
Property | Type | Description |
---|---|---|
"appDefinitionOverrides" |
object |
Valid app definition properties that will override the ones in all app definitions from the app store. Top-level app definition properties can be specified directly in the "appDefinitionOverrides" object. The properties that are found under the "details" top-level key for each app definition type must be specified in a top-level object with the same name as the app type - "window" , "exe" , "node" , "workspaces" , "webGroup" , "clickonce" , "citrix" or "childWindow" . Available since io.Connect Desktop 9.1. |
"details" |
object |
Required. Specific details about the app store. |
"isRequired" |
boolean |
If true (default), the app store will be required. If the app store can't be retrieved, io.Connect Desktop will throw an error and shut down. If false , io.Connect Desktop will initiate normally, without apps from that store. |
"type" |
string |
Required. Type of the app store. Must be set to "rest" for remote app stores. |
The "details"
object for a remote app store has the following properties:
Property | Type | Description |
---|---|---|
"auth" |
string |
Authentication configuration. Can be one of "no-auth" (default), "negotiate" or "kerberos" . |
"cacheFolder" |
string |
Location the persisted configuration files. |
"enablePersistentCache" |
boolean |
If true (default), will cache and persist the configuration files locally (e.g., in case of connection interruptions). |
"pollInterval" |
number |
Interval in milliseconds at which to poll the REST service for updates. Default is 60000 . |
"proxy" |
string |
HTTP proxy to use when fetching data. |
"readCacheAfter" |
number |
Interval in milliseconds after which to try to read the cache. Default is 30000 . |
"rejectUnauthorized" |
boolean |
If true (default), SSL validation will be enabled for the REST server. |
"requestTimeout" |
number |
Timeout in milliseconds to wait for a response from the REST server. Default is 20000 . |
"startRetries" |
number |
Number of times io.Connect Desktop will try to connect to the REST server. Default is 5 . |
"startRetryInterval" |
number |
Interval in milliseconds at which io.Connect Desktop will try to connect to the REST server. Default is 10000 . |
"url" |
string |
Required. The URL to the REST service providing the app definitions. |
The remote store must return app definitions in the following response shape:
{
"applications": [
// List of app definition objects.
{}, {}
]
}
ℹ️ For details on working with remote app stores compliant with FDC3 App Directory standards, see the FDC3 Compliance section and the FDC3 App Directory documentation.
ℹ️ For a reference implementation of a remote app definitions store, see the Node.js REST Config example that implements the FDC3 App Directory and is compatible with io.Connect Desktop. This basic implementation doesn't take the user into account and returns the same set of data for all requests. For instructions on running the sample server on your machine, see the README file in the repository.
ℹ️ For a .NET implementation of a remote app definitions store, see the .NET REST Config example.
io.Manager
You can also use io.Manager for hosting and retrieving app stores. io.Manager is a complete server-side solution for providing data to io.Connect. To configure io.Connect Desktop to fetch app definitions from io.Manager, set the "type"
property of the app store configuration object to "server"
:
{
"appStores": [
{
"type": "server"
}
]
}
The server app store object has the following properties:
Property | Type | Description |
---|---|---|
"appDefinitionOverrides" |
object |
Valid app definition properties that will override the ones in all app definitions from the app store. Top-level app definition properties can be specified directly in the "appDefinitionOverrides" object. The properties that are found under the "details" top-level key for each app definition type must be specified in a top-level object with the same name as the app type - "window" , "exe" , "node" , "workspaces" , "webGroup" , "clickonce" , "citrix" or "childWindow" . Available since io.Connect Desktop 9.1. |
"isRequired" |
boolean |
If true (default), the app store will be required. If the app store can't be retrieved, io.Connect Desktop will throw an error and shut down. If false , io.Connect Desktop will initiate normally, without apps from that store. |
"type" |
string |
Required. Type of the app store. Must be set to "server" for app stores retrieved from io.Manager. |
If you are using only io.Manager for retrieving app definitions, you can set the "appStores"
key to an empty array. io.Connect Desktop will automatically try to connect to io.Manager using its configuration, and will retrieve the app definitions from it, if any.
System App Stores
Besides the regular app stores that usually contain definitions for end user apps, you can define system app stores for critical apps that must be auto started with the platform. The system stores aren't monitored for changes and can be only of type "path"
and "rest"
. To define a system app store, use the "systemAppStores"
top-level key in the system.json
file, which accepts an array of objects defining one or more system app stores.
The following example demonstrates how to configure a local system app store:
{
"systemAppStores": [
{
"type": "path",
"details": {
"path": "./config/system-apps"
}
}
]
}
ℹ️ For details on how to set the priority of system apps, see the Configuration > Application > Boot Sequence section.
App Titles
The titles of io.Connect apps (web and native) can be specified in the app definition. The titles of io.Connect Windows hosting the app instances can be modified programmatically or manually by the user. For web apps, you can instruct io.Connect Desktop to persist the custom titles of their instances when the user saves them in a Layout, and you can also define the behavior for synchronizing the window title with the document title. It's also possible to define a default title format for all io.Connect app instances.
App Definition
The app title set in the app definition will be displayed as an app title in the io.Connect launcher and will be used as a default window title for the instances of native apps, as well as for the instances of web apps that don't already have a document title. To specify an app title, use the "title"
top-level key in the app definition:
{
"title": "My App",
"name": "my-app",
"type": "window",
"details": {
"url": "https://my-app.com"
}
}
Modifying Titles
Users can modify the titles of app instances manually by double clicking on the window title:
The io.Connect APIs enable you to modify window titles programmatically. The following example demonstrates customizing the window title via the io.Connect JavaScript API by using the setTitle()
method of the IOConnectWindow
object:
const myWindow = io.windows.my();
await myWindow.setTitle("New Title");
Persisting Custom Titles
It's possible to instruct io.Connect Desktop to persist the custom titles of web app instances when the user saves their app arrangement in a Layout. To specify global settings for the desired behavior, use the "title"
property of the "saveInLayout"
object under the "windows"
top-level key in the system.json
system configuration file of io.Connect Desktop. To specify settings per app, use the "title"
property of the "saveInLayout"
object under the "details"
top-level key in the app definition. This property accepts either a Boolean value or a list of Layout types for which to persist the custom title. The settings in the app definition will override the global system configuration.
Providing global settings for persisting app titles:
{
"windows": {
"saveInLayout": {
"title": ["Global", "Workspace"]
}
}
}
Overriding the global system settings per app:
{
"details": {
"saveInLayout": {
"title": false
}
}
}
Synchronizing Titles
Titles of web app instances and Workspaces App instances can be synchronized with the document title whenever the document title changes. To specify global settings for the desired behavior, use the "syncTitleWithDocumentTitle"
property of the "windows"
top-level key in the system.json
system configuration file of io.Connect Desktop. To specify settings per app, use the "syncTitleWithDocumentTitle"
property of the "details"
top-level key in the app definition. The settings in the app definition will override the global system configuration.
Providing global settings for synchronizing app titles:
{
"windows": {
"syncTitleWithDocumentTitle": true
}
}
Overriding the global system settings per app:
{
"details": {
"syncTitleWithDocumentTitle": false
}
}
Available since io.Connect Desktop 9.4
The "syncTitleWithDocumentTitle"
property also accepts a "preserveCustomTitle"
string as a value. If set to "preserveCustomTitle"
, the window title will be synchronized with the document title until the moment the user or the API sets a custom title for the window. After that, the custom title will be preserved and the window title won't be synchronized with the document title.
{
"details": {
"syncTitleWithDocumentTitle": "preserveCustomTitle"
}
}
Title Format
To set a format for the titles of app instances, use the "titleFormat"
property of the "applications"
top-level key in the system.json
system configuration file of io.Connect Desktop. The supported macros are {title}
and {instanceIndex}
. The {title}
macro will be substituted with the title set in the app definition and the {instanceIndex}
macro will be substituted with the consecutive number of the started app instance which is incremented for each new app instance starting from 1
. If all instances are closed, the counter is reset. If some instances are closed while others are still running, the counter will continue to increment accordingly:
{
"applications": {
"titleFormat": "{title} ({instanceIndex})"
}
}
⚠️ Note that if the window title is set programmatically via the io.Connect APIs, the specified title format will be overridden. For web apps and Workspaces Apps, the
"syncTitleWithDocumentTitle"
property will override the specified title format.